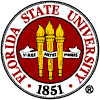
|
COT 5410-01 Fall 2004
Algorithms
Chris Lacher
Notes 8: Set < Binary Tree >
|
BinaryTree
- Node: data + parent, leftChild, rightChild
- Navigator: pointer to Node, operators: ++N (go to left child) ,
N++ (go to right child), --N (go to parent)
BinaryTree::Iterator
- Iterator Data: Navigator
- Iterator Interface: Bidirectional Iterator
- BinaryTree::Begin()
{
Iterator I;
I.Initialize(*this);
return I;
}
BinaryTree::Iterator::Initialize(BinaryTree B)
{
// start at root
N = B.Root();
// then slide left to lowest lchild
while (N.HasLeftChild())
++N;
}
BinaryTree::Iterator operator++()
{
if (!N.Valid())
{
return *this;
}
// now we have a valid navigator
if (N.HasRightChild())
// slide down the left subtree of right child
{
N++;
while (N.HasLeftChild())
++N;
}
else
// back up to first ancestor not already visited
// as long as we are parent's right child, then parent has been visited
{
int NwasRightChild;
do
{
NwasRightChild = N.IsRightChild();
--N;
}
while (NwasRightChild);
}
return *this;
}
Analysis of Iterator Operations
Claim: For a BinaryTree B, the traversal loop
for (I = B.Begin(); I != B.End(); ++I){}
has exactly 2n iterations, where n is the number of nodes in
the tree.
Proof: The traversal crosses each edge of the tree exactly twice, once
descending and once ascending. There is also one initial move to get on the tree
and one final move to get off the tree. Therefore the traversal loop runs
exactly 2e + 2 times, where e = number of edges of B.
Since e = n+ 1, 2e + 2 = 2n, proving the claim.
Corollary: Operator ++() has constant amortized runtime.
BinarySearchTree
- BinaryTree + Total Ordering nodes
- Inherits Navigator, Iterator from BinaryTree
- Search Algorithm
Includes(t)
{
Navigator N = B.Root();
while (N.Valid())
{
if (t.key < N.key)
++N;
else if (N.key < t.key)
N++;
else // t.key == N.key
return N;
}
return N; // null navigator
}
Runtime: O(depth)
Insert
Remove
Animation
Set < BinarySearchTree >
Set::Iterator < BinarySearchTree::Iterator >
- Iterator Interface: Bidirectional Iterator
- Simple Translation