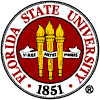
|
COT 5410-01 Fall 2004
Algorithms
Chris Lacher
Notes 10: Graphs and Graph Algorithms
|
Graph Representations
- Adjacency List
- Adjacency Matrix
- Variants:
- Directed Graphs
- Weighted Graphs
- Weighted Digraphs
- Graph as Index
Breadth First Search
Graph in adjacency list representation (vertices indexed 0,1,...n-1)
s = start vertex
Vector < color_type > color // color[v] used in algorithm body
Vector < vertex > parent // parent[v] computed by algorithm
Vector < int > distance // distance[v] = number of edges between start and v by algorithm
Queue < vertex > Q // algorithm control structure
Body
// startup phase
for(each vertex except s)
{
color[v] = white;
distance[v] = infinity;
parent[v] = NIL;
}
color[s] = gray;
distance[s] = 0;
parent[s] = 0;
Q.MakeEmpty();
Q.Push(s);
// search phase
while (!Q.Empty())
{
u = Q.Pop();
for each v in ADJ[u]
{
if (color[v] = white)
{
color[v] = gray;
distance[v] = distance[u] + 1;
parent[v] = u;
Q.Push(v);
}
}
color[u] = black;
}
Analysis
- Q contains exactly the gray vertices
- No vertex is ever changed to white (after initialization)
- Therefore: vertex is pushed at most one, hence popped at most once
- Therefore total time of Queue operations is O(V)
- ADJ[u] is scanned only when u is popped - one time only
- Therefore total time scanning adjacency lists is O(E)
- Therefore runtime of DFS is O(V + E)
Note that size(G) = Θ(V + E), so this is asytmtotically the same
runtime as a traversal
(Re-)Constructing The BFS Tree
BFS Theorem:
- distance[v] < infinity iff v is reachable from s
- distance[v] is the length of a shortest path from s to v
- If v is reachable from s, the BFS tree path from s to v is a shortest path from s to v
BFS on DiGraphs
Depth First Search
Graph or Digraph in adjacency list representation (vertices indexed 0,1,...n-1)
s = start vertex
Vector < color_type > color // color[v] used in algorithm body
Vector < vertex > parent // parent[v] computed by algorithm
Vector < int > d // d[v] = discovery time - when color changes to gray
Vector < int > f // f[v] = finish time - when color color changes to black
Stack < vertex > Q // algorithm control structure
unsigned int time // increments at each color change
Body
// startup phase
for(each vertex v)
{
color[v] = white;
parent[v] = NIL;
}
time = 0;
// search phase - one vertex s
if (color[s] == white)
color[s] = gray;
parent[s] = 0;
++time;
d[s] = time;
Q.MakeEmpty();
Q.Push(s);
while (!Q.Empty())
{
u = Q.Top();
if (v in ADJ[u] && color[v] == white)
{
color[v] = gray;
++ time;
d[v] = time;
parent[v] = u;
Q.Push(v);
}
else
{
color[u] = black;
++time;
Q.Pop();
f[u] = time;
}
}
Analysis
- Q contains exactly the gray vertices
- No vertex is ever changed to white (after initialization)
- Therefore: vertex is pushed at most one, hence popped at most once
- Therefore total time of Queue operations is O(V)
- ADJ[u] is scanned only when u is popped - one time only
- Therefore total time scanning adjacency lists is O(E)
- Therefore runtime of DFS is O(V + E)
Note that size(G) = Θ(V + E), so this is asytmtotically the same
runtime as a traversal
(Re-)Constructing The DFS Forrest
DFS Theorem:
- d[v] is time v is pushed onto the stack
- f[v] is time v is popped off of the stack
- For a vertex v let I(v) = [d[v], f[v], the interval of
time that v is gray (on the stack). Then for any u,v, exactly
one of these statements holds:
- I(u) and I(v) are disjoint: u and v
are not in the same tree of the DFS forrest
- I(u) is a subset of I(v): u is a descendant of v
in the DFS forrest
- I(u) is a superset of I(v): u is an ancester of v
in the DFS forrest
- Vertex v is a proper descendant of u in the DFS forrest iff at
time d[u], v is reachable from u along a path of
white vertices.
Four edge types:
- Tree Edges: Edges in the DFS forrest
- Back Edges: Edges from a vertex to an ancester in the DFS forrest
- Forward Edges: Non-tree edges from a vertex to a descendant in the
DFS forrest
- Cross Edges: All other edges
Modify DFS to classify edges as vertices are discovered
- to white vertex ==> tree edge
- to gray vertex ==> back edge
- to black vertex ==> forward or cross edge
After DFS of an undirected graph, there are no forward or cross edges
Topological Sort
- Directed Acyclic Graph (DAG)
- Theorem: Digraph G is a DAG iff DFS yields no back edges
- Topological Sort Algorithm:
- Perform DFS
- EnQueue vertices in finishing order
- Top Sort Theorem: After Top Sort Alg, Output Queue contains all vertices iff
Minimum Spanning Trees
set A; // build up the MST here
A.MakeEmpty()
while (A is not spanning tree)
{
find safe edge e for A
A.Insert(e)
return A
}
If A is a subset of a MST for (G,w), a safe edge for
A is an edge that A.Insert(e) is a subset of a MST for (G,w).
Kruskal: greedy algorithm with A a MSForrest
Prim: greedy algorithm with A a MSTree
Single-Source Shortest Paths
All-Pairs Shortest Paths