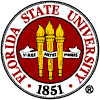
|
COT 5405
Advanced Algorithms
Chris Lacher
Notes 2: Recurrences
|
Concepts
- Recurrence
- Function f(n) defined for n = 0, 1, 2, ... (or some other initial
segment of integers)
- Equation or inequality that describes f(n) in terms of
f(k), k < n
- Examples
f(n) = f(n-1) + f(n-2) // defines Fibanacci sequence
g(n) = 2g(n/2) + n // uses integer arithmetic
Initial conditions
- Required values for first few inputs
- Examples:
f(0) = 0, f(1) = 1 // Fibanacci
may be unspecified
Exact Solution
- Find (set of all) function(s) f(n) satisfying the recurrence
(and the ICs)
- Example: Fibanacci
f(n) = (φ1n -
φ2n) ⁄ √5
Asymptotic Solution
- Example:
g(n) ≤ O( n log n )
Linear Recurrences, Polynomial Method
- Linear recurrence of order k:
f(n) =
a1f(n - 1) +
a2f(n - 2) + ... +
akf(n - k), where the coefficients
ak are constants
- Characteristic polynomial:
P(x) =
xk -
a1xk-1 -
a2xk-2 - ... -
ak
- Characteristic equation:
P(x) = 0 , or
xk =
a1xk-1 +
a2xk-2 + ... +
ak
- Roots of characteristic polynomial are solutions to recurrence
- If r1 and r2 are roots then
f(n) = c1r1n +
c2r2n is a solution for any constants
c1 and c2 .
- Degree two case:
- f(n) =
a1f(n - 1) +
a2f(n - 2)
-
Suppose r2 - a1r -
a2 has two distinct roots r1 and
r2.
Then the sequence {fn} is a
solution of the recurrence iff
fn = c1r1n
+ c2r2n for n = 0, 1, ...,
where c1 and c2 are constants.
-
Suppose r2 - a1r -
a2 has only one root r0.
Then the sequence {fn} is a solution of the recurrence
iff
fn = c1r0n
+ c2nr0n for n = 0, 1, ...,
where c1 and c2 are constants.
- General degree n case - mutatis mutandis
- Example: Fibanacci
Techniques for non-linear cases
- Substitution
- Recursion Tree
- Master Theorem.
- Hypotheses:
- a >= 1 and b > 1 are constants
- f(n) is a function defined for the non-negative integers
- T(n) satisfies the recurrence T(n) =
aT(n/b) + f(n)
[divide-and-conquer recurrence]
- Conclusions:
-
If f(n) <= O(nlogba
- ε) for some constant ε > 0, then
T(n) = Θ(nlogba).
-
If f(n) = Θ(nlogba),
then
T(n) = Θ(nlogba
lg n).
-
If f(n) >= Ω(nlogba
+ ε) for some constant ε > 0, and
if af(n/b) <= cf(n) for some constant c
< 1 and large n,
then
T(n) = Θ(f(n)).
The Master Theorem gives information about the relative asymptotic dominance of the
"divide" term aT(n/b)
versus the "overhead" term f(n). Note that
- p(n) = nlogba is a
solution to the pure recurrence
P(n) = aP(n/b)
as shown by substitution:
ap(n/b)
= a(n/b)logba
= a(nlogba / blogba)
= a(nlogba / a)
= nlogba
= p(n)
- Thus we can paraphrase the Master Theorem as follows:
- When the non-recursive term f(n) is asymptotically dominated by something strictly slower
growing than the pure recurrence P(n), then L(n) and p(n)
are asymptotically the same.
-
When f(n) is asymptotically equivalent to P(n), then
L(n) is asymptocially equivalent to p(n) multiplied by log n.
(This happens because log n is the depth of the recursion tree.)
-
When f(n) asymptotically dominates something strictly faster
growing than P(n), and f(n) is sub-recursive,
then
L(n) is asymptocially equivalent to f(n).
The master theorem has somewhat intricate hypotheses. Yet it is applicable to a
sizeable portion of algorithms of the divide and conquer design, wherein
a computation is reduced to a recursive call on a smaller input set plus an
overhead term.