Functions 1: Predefined and Value-Returning Functions
What are Functions?
In general, we use (call) functions (aka: modules, methods, procedures, subprocedures, or subprograms) to perform a specific (atomic) task. In algebra, a function is defined as a rule or correspondence between values, called the function's arguments, and the unique value of the function associated with the arguments. For example:
If f(x) = 2x + 5, then f(1) = 7, f(2) = 9, and f(3) = 11
1, 2, and 3 are arguments
7, 9, and 11 are the corresponding values
Bjarne Stroustrup's C++ Glossary
- Functions are building blocks
- Allow complicated programs to be divided into manageable components
- Called modules, methods, procedures, or sub-procedures
- Like miniature programs
- Can be put together to form larger program
- Some advantages of functions:
- Programmer can focus on just the function: develop it, debug it, and test it
- Various developers can work on different functions simultaneously
- Can be used in more than one place in a program--or in different programs
Predefined Functions
Using predefined functions:
- Some predefined C++ mathematical functions:
- pow(x,y)
- sqrt(x)
- floor(x)
- Predefined functions are organized into separate libraries
- C++ Standard Library contains many predefined functions to perform various operations
- I/O functions are in iostream header
- Math functions are in cmath header
- Power Function - pow(x,y):
- Power function pow(x,y) has two parameters
- pow(x,y) returns value of type double
- pow(x,y) calculates x to the power of y: pow(2,3) = 8.0
- x and y called parameters (or arguments) of function pow
- Square Root Function - sqrt(x):
- Square root function sqrt(x) has only one parameter
- sqrt(x) returns value of type double
- sqrt(x) calculates non-negative square root of x, for x >= 0.0: sqrt(2.25) = 1.5
- Floor Function - floor(x):
- Floor function floor(x) has only one parameter
- floor(x) returns value of type double
- floor(x) calculates largest whole number not greater than x: floor(48.79) = 48.0
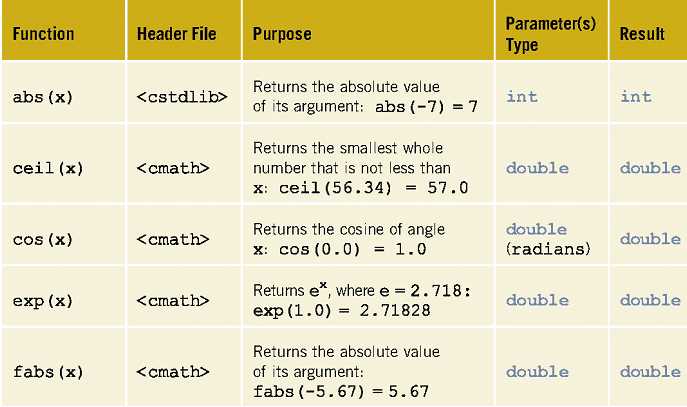
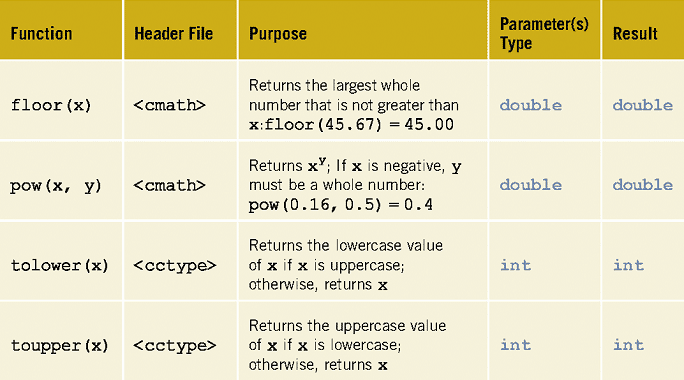
C++ Standard Library reference
Rogue Wave C++ Standard Library Class Reference
Microsoft MSDN Library - Standard C++ Library Reference
Microsoft MSDN Library - C/C++ Languages
User-Defined Functions
Using User-Defined functions:
- Two types:
- Void functions (nonvalue-returning): no return type, do not return a value
- Value-returning functions: have a data type, return only one value to caller
- When utilizing functions:
- Include correct header file
- Know function name
- Know number of parameters, if any
- Know data type of parameters
- Know data type of value computed by function
- Value-returning function used 3 ways:
- Assignment statement
- Output statement
- Argument (actual parameter) in another function call
- Creating functions:
Mnemonic: "ProDeCall":
- Prototype
- Definition
- Call
Function definition includes:
- Header (or heading) includes:
- Function name
- Number of parameters (if any)
- Data type of each parameter
- Type of function (data type or void)
- Body includes:
- Code to accomplish task
- ***Any variables (if any) declared in body of function are local to function
Function prototype includes:
Function call includes:
- Name with actual parameters (if any) in parentheses
- In call statement, specify only arguments (actual parameters), not data types
- One-to-one correspondence between actual and formal parameters
Mnemonic: "TON" - (must agree in Type, Order, and Number)
- Value-returning function called in an expression (only 3 ways):
- Assignment statement
- Output statement
- Argument (actual parameter) in another function call
- Function call results in execution of body of called function
//function call syntax:
x = functionName(actual parameter list); //Assignment, Output, Argument in another function call
- Call to value-returning function with empty formal parameter list:
x = functionName(); //Assignment, Output, Argument in another function call
- After value-returning function is invoked, function returns value via return statement
//return statement syntax:
return expression;
- When return statement executes:
- Function terminates
- Control goes back to caller
- ***When return statement executes in function main(), program terminates
Flow of Execution
Program Execution:
- Begins with first statement in function main()
- Additional functions invoked when called
- Function prototypes appear before function definitions--that is, before function main()
- Compiler translates prototypes first
- Function call invocation:
- Transfer of control to first statement in body of called function
- After function body executed: control passed back to point immediately following function call
- Value-returning function returns value
- After function execution: value that function returns replaces function call statement
Function Example:
/*
This program illustrates value-returning functions
and compares two, then three numbers.
written by Mark K. Jowett, Ph.D.
6:03 PM 9/11/2004
*/
#include<iostream>
//using namespace std;
//function prototypes
double testTwo(double x, double y);
double testThree(double x, double y, double z);
int main()
{
double num1, num2;
//value-returning function used in output
std::cout << "The larger of 5 and 10 is "
<< testTwo(5, 10) << std::endl; //function call
std::cout << "Enter one number: ";
std::cin >> num1;
std::cout << "Enter another number: ";
std::cin >> num2;
std::cout << std::endl;
//value-returning function used in output
std::cout << "The larger of " << num1 << " and "
<< num2 << " is " << testTwo(num1, num2) << std::endl; //function call
//value-returning function used in output
std::cout << "The largest of 10, 15, and 20 is "
<< testThree(10, 15, 20) << std::endl; //function call
std::cout << "Press Enter key to exit...";
std::cin.get(); std::cin.get(); // make DOS window stay open
return 0;
}
//function definition
double testTwo(double x, double y)
{
if (x >= y)
return x;
else
return y;
}
//function definition
double testThree (double x, double y, double z)
{
//value-returning function used as argument in another function call
return testTwo(x, testTwo(y, z));
}
Displays:
The larger of 5 and 10 is 10
Enter one number: 2
Enter another number: 3
The larger of 2 and 3 is 3
The largest of 10, 15, and 20 is 20
Press Enter key to exit...